Learning Cairo - The Syntax of Structures (8)
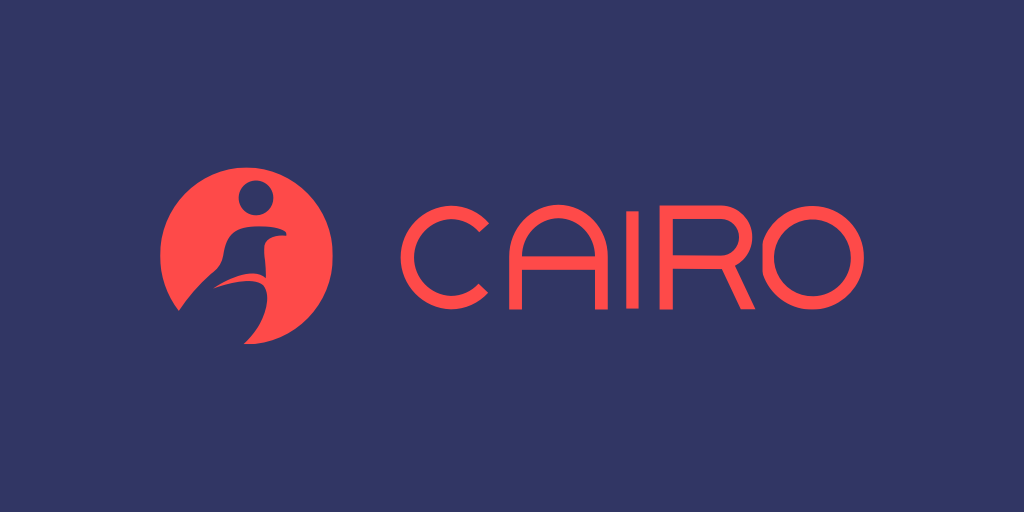
Prerequisite
Install cairo-run.
Structures
Cairo also has the concept of structures. In previous articles, we have already used some structures. In this article, we will study the syntax of structures in detail.
Similar to Solidity, we can use the struct
keyword to define a structure. For example:
#[derive(Copy, Drop)]
struct User {
active: bool,
username: felt252,
email: felt252,
sign_in_count: u64,
}
Once the structure is defined, we can create an instance of it, for example:
#[derive(Copy, Drop)]
struct User {
active: bool,
username: felt252,
email: felt252,
sign_in_count: u64,
}
fn main() {
let user1 = User {
active: true, username: 'someusername123', email: '[email protected]', sign_in_count: 1
};
}
When creating an instance, you need to set the value for each field in the structure. The order of the fields doesn't need to be the same as defined in the structure, which means the following syntax is also acceptable:
fn main() {
let user1 = User {
username: 'someusername123', active: true, sign_in_count: 1, email: '[email protected]'
};
}
We can read the fields in the structure using dot notation. For instance: user1.username
, user1.active
. If the instance itself is mutable, we can also assign values to its fields using dot notation, such as:
fn main() {
let mut user1 = User {
active: true, username: 'someusername123', email: '[email protected]', sign_in_count: 1
};
user1.email = '[email protected]';
}
Cairo does not allow a particular field in a structure to be mutable, so if you want to define a mutable instance, you have to define the entire instance as mutable.
Remember how we mentioned in previous articles that by placing an expression at the end of a function, we can omit writing the return
statement? For structures, we can use this method to construct a structure instance, for instance:
fn build_user(email: felt252, username: felt252) -> User {
User { active: true, username: username, email: email, sign_in_count: 1, }
}
The build_user
function takes email
and username
fields and returns a structure of type User
. We can directly construct a structure instance and remember to omit the semicolon, so the build_user
function will return a structure of type User
.
We noticed in the example above that the username
field is assigned the username
variable, and the email
field is assigned the email
variable. This seems a bit tedious, but fortunately, Cairo provides us with a way to simplify it.
For fields where the field name and variable name are the same, we can omit one of them. For instance, the above build_user
function can be rewritten as:
fn build_user(email: felt252, username: felt252) -> User {
User { active: true, username, email, sign_in_count: 1, }
}
This shorthand means that we assign the username
variable to the username
field and assign the email
variable to the email
field.
Changing the order of field assignments is also possible:
fn build_user(email: felt252, username: felt252) -> User {
User { email, username, sign_in_count: 1, active: true }
}
Conclusion
We can use struct
to define structures and use dot notation to access their field values. When creating a structure instance, if the field name and variable name are the same, one can be omitted, saving effort.
Low latency and free Starknet node awaits!
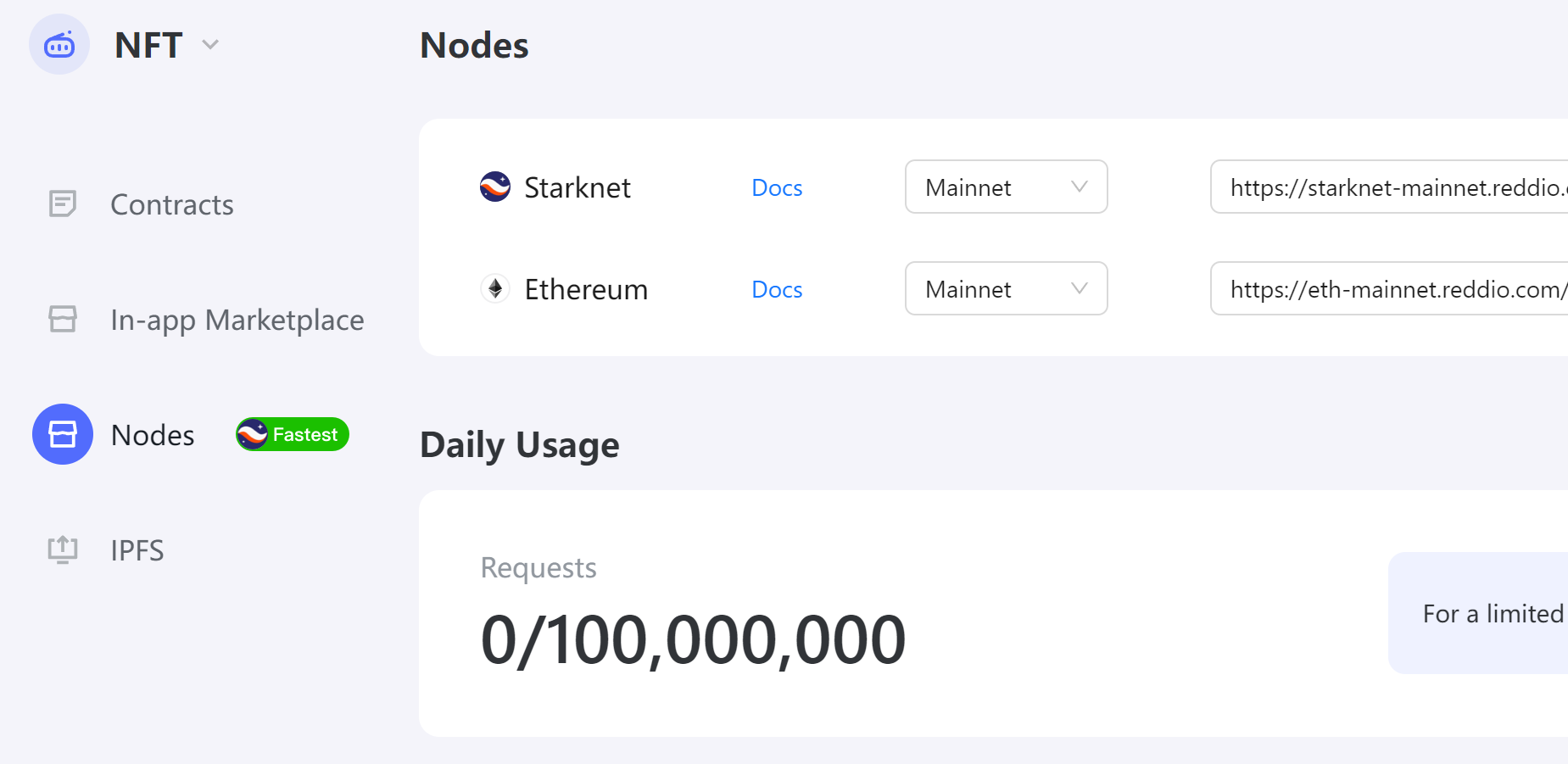
For a limited time, Reddio is offering unrestricted access to its high-speed StarkNet Node, completely free of charge. This is an unparalleled opportunity to experience the fastest connection with the lowest delay. All you need to do is register an account on Reddio at https://dashboard.reddio.com/ and start exploring the limitless possibilities.
You can discover why Reddio claims the fastest connection by reading more here.