Learning Cairo - Control Flows (4)
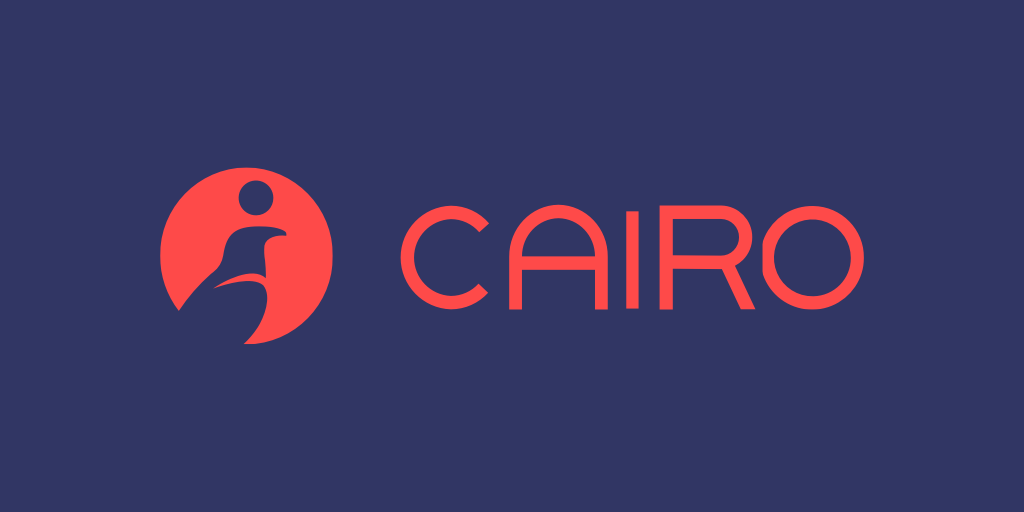
Prerequisite
Install cairo-run.
Control Flow
Today, we will learn about control flow in Cairo. The most common control flow expressions are if
and loop
.
if
Expression
The if
statement in Cairo is similar to Solidity, with the difference that Cairo’s if
condition does not require parentheses. For example:
use debug::PrintTrait;
fn main() {
let a = 1;
if a == 5 {
'condition was true'.print();
} else {
'condition was false'.print();
}
}
In Cairo, there are no parentheses around the condition a == 5
. In Solidity, parentheses would be required:
function condition() public {
uint256 a = 1;
if (a == 1) {
console.log("condition was true");
} else {
console.log("condition was false");
}
}
Running the above Cairo code produces the expected result:
[DEBUG] condition was false (raw: 2217480802923670243215595364183720060804428645)
Run completed successfully, returning []
Using if
in a let
Statement
Since if
is an expression, based on what we have learned about expressions, we can use if
expressions to assign values to variables. Let’s see an example:
use debug::PrintTrait;
fn main() {
let cool = if 3_u8 > 2_u8 {
true
} else {
false
};
cool.print();
}
In this example, the if
expression first evaluates the value of 3_u8 > 2_u8
. If it’s true
, it returns true
; otherwise, it returns false
. Finally, the returned value is assigned to the variable cool
.
Loops
Cairo provides loop functionality, but unlike other languages, it doesn’t have common loop keywords like for
or while
. In Cairo, we use the loop
keyword for loops. Let’s see an example:
use debug::PrintTrait;
fn main() {
let mut i:usize = 0;
loop {
if i > 10 {
break();
}
'again!'.print();
}
}
In this example, the loop
is the loop body. Since i
never changes, i > 10
will always be false
, resulting in again!
being printed continuously.
Note that when running this code, you need to specify a gas limit, as the code contains a loop. Cairo requires a gas limit to prevent infinite loops, similar to the mechanism in Solidity. In Solidity, it is also possible to write code with an infinite loop, but due to the gas limit, even if it is an infinite loop, the program will eventually terminate to prevent attacks on the Ethereum network.
When running Cairo code with a loop, you need to add a gas limit to the command to specify the amount of gas allocated to the code:
--available-gas=20000000
Let’s compile and run the above Cairo code:
....
....
[DEBUG] again! (raw: 107096643825185)
[DEBUG] again! (raw: 107096643825185)
[DEBUG] again! (raw: 107096643825185)
Run panicked with [375233589013918064796019 ('Out of gas'), ].
Remaining gas: 410
As you can see, again!
is printed multiple times, but eventually the program terminates due to insufficient gas, which is what we expected.
To correct the code, we simply need to ensure that the exit condition is met. We can achieve this by adding i += 1;
inside the loop body:
use debug::PrintTrait;
fn main() {
let mut i:usize = 0;
loop {
if i > 10 {
break();
}
i += 1;
'again!'.print();
}
}
Loop with Return Value
Similar to if
, loop
can also return a value. Let’s see an example:
use debug::PrintTrait;
fn main() {
let mut counter = 0;
let result = loop {
if counter == 10 {
break counter * 2;
}
counter += 1;
};
'The result is '.print();
result.print();
}
In the loop body, we can use break
to return a value. In the example above, break counter * 2;
returns the value of counter * 2
from the loop.
Summary
In this section, we learned about the basic control flow statements in Cairo, including if
and loop
. Unlike other languages, Cairo does not have for
or while
loop statements, but only the loop
construct.
You can star our repository, we will closely update it alongside StarkNet rolls out 1.0 fully, or join our Discord if you have any questions or want to contribute.
Call out StarkNet Beta Testers!
Reddio is building developer tools for StarkNet to help you accelerate the process to develop StarkNet applications. We are inviting all of StarkNet developers to join our beta testing group, try out brand-new features and tell us what you think.
https://share.hsforms.com/1E88oQkqMSJifUV1CqR_WrQd30xn
Low latency and free Starknet node awaits!
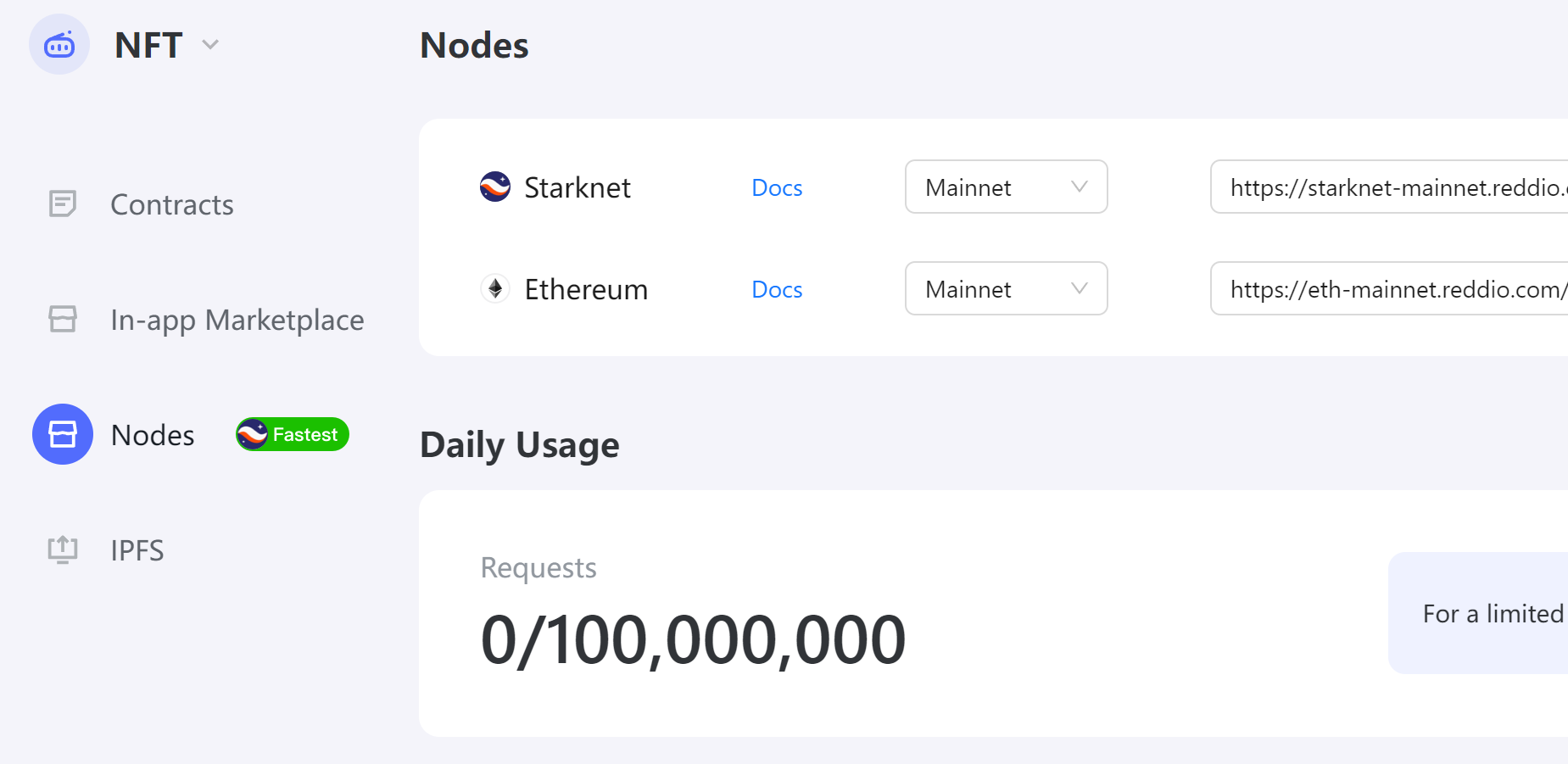
For a limited time, Reddio is offering unrestricted access to its high-speed StarkNet Node, completely free of charge. This is an unparalleled opportunity to experience the fastest connection with the lowest delay. All you need to do is register an account on Reddio at https://dashboard.reddio.com/ and start exploring the limitless possibilities.
You can discover why Reddio claims the fastest connection by reading more here.